The n-queens puzzle is the problem of placing n
queens on an n x n
chessboard such that no two queens attack each other.
Given an integer n
, return all distinct solutions to the n-queens puzzle. You may return the answer in any order.
Each solution contains a distinct board configuration of the n-queens' placement, where 'Q'
and '.'
both indicate a queen and an empty space, respectively.
Example 1:
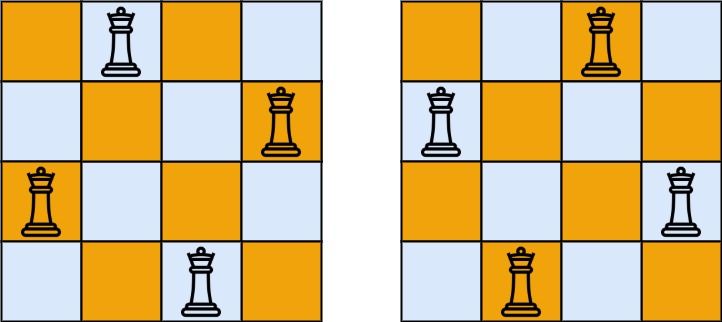
Input: n = 4 Output: [[".Q..","...Q","Q...","..Q."],["..Q.","Q...","...Q",".Q.."]] Explanation: There exist two distinct solutions to the 4-queens puzzle as shown above
Example 2:
Input: n = 1 Output: [["Q"]]
Constraints:
1 <= n <= 9
--------------------2 nd pass----------------------
DFS ---------- Use 2D array
出现的问题,还是在于,测试diagonal的时候, 两条diagonal的表达有问题。
并不是对角线上的2条才需要检查。 左右的diagonal方向都需要。
class Solution {public:vector<vector<string>> solveNQueens(int n) {vector<vector<char>> board(n, vector<char>(n, '.'));vector<vector<string>> res;dfs(board, res, 0);return res;}private:void dfs(vector<vector<char>>& board, vector<vector<string>>& res, int row) {int n = board.size();if (row == n) {vector<string> curRes;for (int i = 0; i < n; i++) {string tmp(board[i].begin(), board[i].end());curRes.push_back(tmp);}res.push_back(curRes);} else {for (int j = 0; j < n; j++) {board[row][j] = 'Q';if (isValid(board, row, j))dfs(board, res, row + 1);board[row][j] = '.';}}}bool isValid(vector<vector<char>>& board, int row, int col) {int n = board.size();// check each row ifor (int j = 0; j < n; j++) {if (j != col && board[row][j] == 'Q')return false;}// now check colum : colfor (int i = 0; i < n; i++) {if (i != row && board[i][col] == 'Q')return false;}// check diagonalfor (int i = 0; i < n; i++) {int j = i + (col - row);if (j >= 0 && j < n && i != row && board[i][j] == 'Q')return false;}// check reverse diagonalfor (int i = 0; i < n; i++) {int j = (col + row) - i;if (j >= 0 && j < n && i != row && board[i][j] == 'Q')return false;}return true;}};
--------------3rd pass---------------------
这里用了2D array, 如果用一维数组表示的话(类似于N-Queen II) ,
方法是:一维数组的下标表示横坐标(哪一行),而数组的值表示纵坐标(哪一列)。
Mistakes:
No comments:
Post a Comment