The demons had captured the princess and imprisoned her in the bottom-right corner of a dungeon
. The dungeon
consists of m x n
rooms laid out in a 2D grid. Our valiant knight was initially positioned in the top-left room and must fight his way through dungeon
to rescue the princess.
The knight has an initial health point represented by a positive integer. If at any point his health point drops to 0
or below, he dies immediately.
Some of the rooms are guarded by demons (represented by negative integers), so the knight loses health upon entering these rooms; other rooms are either empty (represented as 0) or contain magic orbs that increase the knight's health (represented by positive integers).
To reach the princess as quickly as possible, the knight decides to move only rightward or downward in each step.
Return the knight's minimum initial health so that he can rescue the princess.
Note that any room can contain threats or power-ups, even the first room the knight enters and the bottom-right room where the princess is imprisoned.
Example 1:
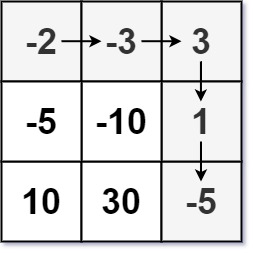
Input: dungeon = [[-2,-3,3],[-5,-10,1],[10,30,-5]] Output: 7 Explanation: The initial health of the knight must be at least 7 if he follows the optimal path: RIGHT-> RIGHT -> DOWN -> DOWN.
Example 2:
Input: dungeon = [[0]] Output: 1
Constraints:
m == dungeon.length
n == dungeon[i].length
1 <= m, n <= 200
-1000 <= dungeon[i][j] <= 1000
A:
就是简单的2D , 但是,这里要 backward calculating
每个A[i][j] 表示的是这个位置需要多少engergy进来。但是由于前面的位置不允许出来的engergy<=0 ---------》 A[i][j] >= 1
比较tricky的是: 要注意每次出去的生命值不能 <= 0 , aka, 每个位置剩余的生命必须 > 0
直接在dungeon[][]上面改,没有用额外的空间. 记录该点 entering的最少的要求
上面的是有问题的。 在于如果没有右边或者左边的时候,我们就不能往那边走class Solution {public:int calculateMinimumHP(vector<vector<int>>& dungeon) {int m = dungeon.size(), n = dungeon[0].size();int rightNeed = 0, downNeed = 0;// use dungeon in place, to mark the min possible// entering_life for [i][j] so it can safely go right&downfor (int i = m-1; i >=0 ; i--) {for (int j = n-1 ; j >=0 ; j--) {rightNeed = (j == n-1) ? 1 : dungeon[i][j+1];downNeed = (i == m-1)? 1 : dungeon[i+1][j];// entering_life = (at least 1)// entering_life + cur_value >= max( right_left_life , down_left_life)dungeon[i][j] = max(1, min( rightNeed, downNeed) - dungeon[i][j] );}}return dungeon[0][0];}};
Mistakes:
1: 一开始A[i][j]的定义不清楚。
2: 忘了检查A[i][j] >0