Q:
Given the head
of a singly linked list, reverse the list, and return the reversed list.
Example 1:
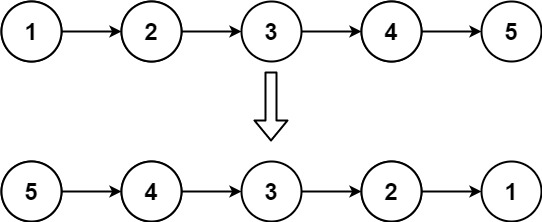
Input: head = [1,2,3,4,5] Output: [5,4,3,2,1]
Example 2:
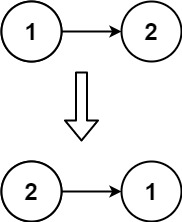
Input: head = [1,2] Output: [2,1]
Example 3:
Input: head = [] Output: []
Constraints:
- The number of nodes in the list is the range
[0, 5000]
. -5000 <= Node.val <= 5000
Follow up: A linked list can be reversed either iteratively or recursively. Could you implement both?
A:
/*** Definition for singly-linked list.* struct ListNode {* int val;* ListNode *next;* ListNode() : val(0), next(nullptr) {}* ListNode(int x) : val(x), next(nullptr) {}* ListNode(int x, ListNode *next) : val(x), next(next) {}* };*/用dummy headerclass Solution {public:ListNode* reverseList(ListNode* head) {ListNode header;while (head) {auto tmp = head;head = head->next;tmp->next = header.next;header.next = tmp;}return header.next;}};或者把 新的head,不用 dummy header, 而是指向最新的head. 只是尽量晚赋值class Solution {public:ListNode* reverseList(ListNode* head) {ListNode* newHead = nullptr;while (head) {ListNode* nextNode = head->next;head->next = newHead;newHead = head;head = nextNode;}return newHead;}};**********Mistakes*************
ListNode *header(0); ---------> this grammar is WRONG.
It initializes
header
as a null pointer (nullptr
) because:
header
is a pointer (ListNode*
).0
is an integer literal, which implicitly converts tonullptr
in C++ when assigned to a pointer.Thus header is equivalent toListNode* header = nullptr; // Explicitly setting it to nullptrThis means
header->next
is an invalid operation becauseheader
isnullptr
.
Correct Way to Create a Dummy Node
If you meant to create an actual
ListNode
object, you should write:
or use dynamic allocation:
ListNode* header = new ListNode(0); // Allocates a real ListNode on the heap