Given a binary tree, find its minimum depth.
The minimum depth is the number of nodes along the shortest path from the root node down to the nearest leaf node.
Note: A leaf is a node with no children.
Example 1:
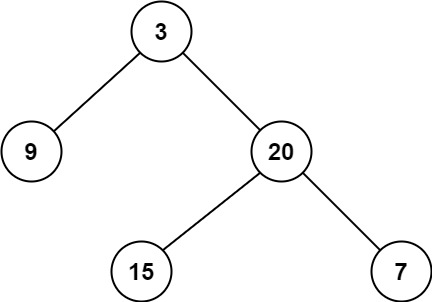
Input: root = [3,9,20,null,null,15,7] Output: 2
Example 2:
Input: root = [2,null,3,null,4,null,5,null,6] Output: 5
Constraints:
- The number of nodes in the tree is in the range
[0, 105]
. -1000 <= Node.val <= 1000
A:
/*** Definition for a binary tree node.* struct TreeNode {* int val;* TreeNode *left;* TreeNode *right;* TreeNode() : val(0), left(nullptr), right(nullptr) {}* TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}* TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left), right(right) {}* };*/class Solution {public:int minDepth(TreeNode* root) {if(!root)return 0;if(! root->left && ! root->right){return 1;}else if( ! root->left){return 1+ minDepth(root->right);}else if( ! root->right){return 1 + minDepth(root->left);}return 1 + min(minDepth(root->left), minDepth(root->right));}};
Mistakes:
1:
注意,这里的depth,不是我们通常所理解的(edge的条数)而是node的数量。
我们设了root==null时, depth =-1. 这样,为了不考虑root.left == null,(或者root.right==null)时的情况。
但是,当root真为空的时候,depth就返回了-1,而不是0了。
2: 注意,题目里,是到nearest left node. 因此,当输入是{1,2}的时候,我们不能考虑
-------------------第二遍-----------------题目理解错误, 要求是nearest leaf node ---------而若一个节点,有左,或者右 child的话,是不能算的。
No comments:
Post a Comment