Given the roots of two binary trees p
and q
, write a function to check if they are the same or not.
Two binary trees are considered the same if they are structurally identical, and the nodes have the same value.
Example 1:
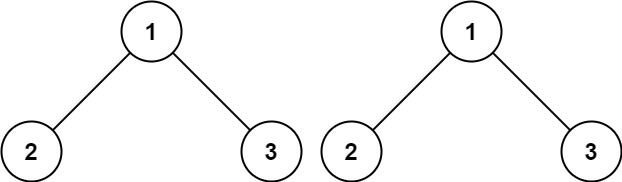
Input: p = [1,2,3], q = [1,2,3] Output: true
Example 2:
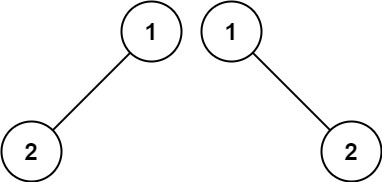
Input: p = [1,2], q = [1,null,2] Output: false
Example 3:
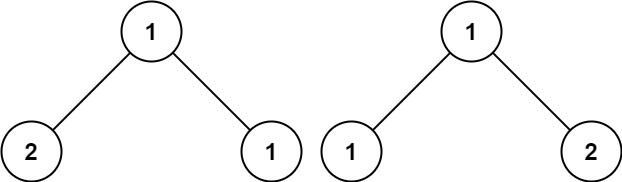
Input: p = [1,2,1], q = [1,1,2] Output: false
Constraints:
- The number of nodes in both trees is in the range
[0, 100]
. -104 <= Node.val <= 104
A:
public class Solution { public boolean isSameTree(TreeNode p, TreeNode q) { if(p == null && q == null){ return true; }else if(p!= null && q == null){ return false; }else if(p == null && q!= null){ return false; }else{ //both p and q are not null if (p.val != q.val ){ return false; }else{ boolean isLeftSame = isSameTree(p.left, q.left); boolean isRightSame = isSameTree(p.right,q.right); return (isLeftSame && isRightSame); } } } }
---------------------------第二遍 --------------------
思路一样,就是 没有用那么多的else if 语句。 因为,都是一个个的情况排除了。
public class Solution { public boolean isSameTree(TreeNode p, TreeNode q) { if(p == null && q == null ) return true; if( ( p== null && q != null ) || (p != null && q==null)) return false; // now both are not null if( p.val != q.val) return false; // not both are the same value boolean left = isSameTree(p.left, q.left); boolean right = isSameTree(p.right, q.right); return left && right; } }
Mistakes:
Learned:
No comments:
Post a Comment