Q:
Given a linked list, determine if it has a cycle in it.
To represent a cycle in the given linked list, we use an integer
pos
which represents the position (0-indexed) in the linked list where tail connects to. If pos
is -1
, then there is no cycle in the linked list.
Example 1:
Input: head = [3,2,0,-4], pos = 1 Output: true Explanation: There is a cycle in the linked list, where tail connects to the second node.
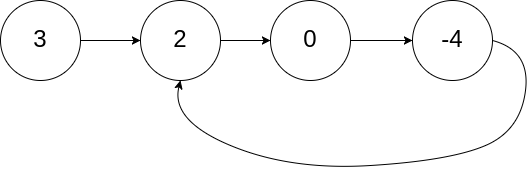
Example 2:
Input: head = [1,2], pos = 0 Output: true Explanation: There is a cycle in the linked list, where tail connects to the first node.
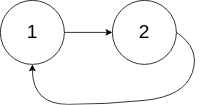
Example 3:
Input: head = [1], pos = -1 Output: false Explanation: There is no cycle in the linked list.

A:
two pointer techniquefollow up: without extra space/** * Definition for singly-linked list. * struct ListNode { * int val; * ListNode *next; * ListNode(int x) : val(x), next(NULL) {} * }; */ class Solution { public: bool hasCycle(ListNode *head) { if(!head) return false; auto slow = head; auto fast = head->next; while(fast && slow!=fast ) { slow = slow->next; fast = fast->next; if(fast) fast = fast->next; } return fast==slow; } };
那就用已给的空间了。 例如用head节点(和head.next) 可是,如何防止被我们变换的node不是重复节点呢?
“you could record the ith(i = 1,2,4,8..........) ListNode* and compare it to your iterating ListNode*"
Mistakes:
Learned:
No comments:
Post a Comment