Given a root node reference of a BST and a key, delete the node with the given key in the BST. Return the root node reference (possibly updated) of the BST.
Basically, the deletion can be divided into two stages:
- Search for a node to remove.
- If the node is found, delete the node.
Follow up: Can you solve it with time complexity O(height of tree)
?
Example 1:
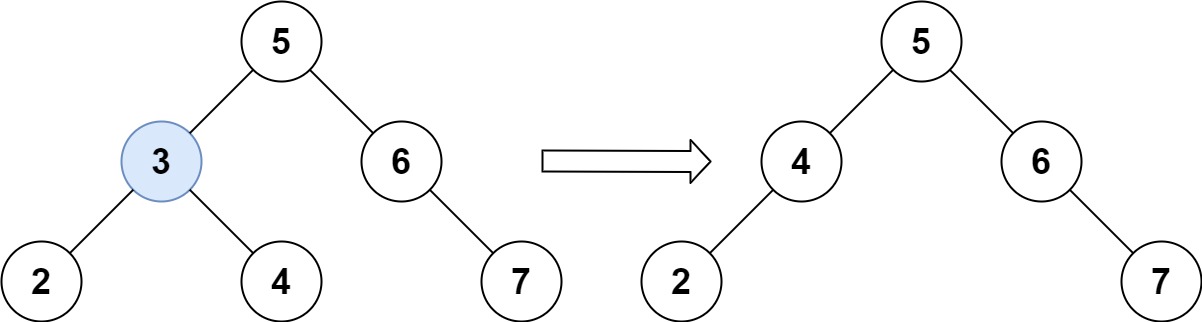
Input: root = [5,3,6,2,4,null,7], key = 3 Output: [5,4,6,2,null,null,7] Explanation: Given key to delete is 3. So we find the node with value 3 and delete it. One valid answer is [5,4,6,2,null,null,7], shown in the above BST. Please notice that another valid answer is [5,2,6,null,4,null,7] and it's also accepted.![]()
Example 2:
Input: root = [5,3,6,2,4,null,7], key = 0 Output: [5,3,6,2,4,null,7] Explanation: The tree does not contain a node with value = 0.
Example 3:
Input: root = [], key = 0 Output: []
Constraints:
- The number of nodes in the tree is in the range
[0, 104]
. -105 <= Node.val <= 105
- Each node has a unique value.
root
is a valid binary search tree.-105 <= key <= 105
A:
**************递归实现********************
/** * Definition for a binary tree node. * struct TreeNode { * int val; * TreeNode *left; * TreeNode *right; * TreeNode() : val(0), left(nullptr), right(nullptr) {} * TreeNode(int x) : val(x), left(nullptr), right(nullptr) {} * TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left), right(right) {} * }; */class Solution {public: TreeNode* deleteNode(TreeNode* root, int key) { TreeNode* parent = new TreeNode(0); parent->left = root; helper(root, parent, true, key); return parent->left; }
private: void helper(TreeNode* root, TreeNode* parent, bool isRootLeftChild, int key) { if (!root) return; if (root->val == key) { // copy node, and remove the most if (root->left) { // if left has value. copy its right most value, // and then remove that value auto runner = root->left; while (runner->right) { runner = runner->right; } root->val = runner->val; helper(root->left, root, true, root->val); // continue to remvoe the copied value } else if (root->right) { auto runner = root->right; while (runner->left) { runner = runner->left; } root->val = runner->val; helper(root->right, root, false, root->val); } else { // no child, we can simply delete this node if (isRootLeftChild) { parent->left = nullptr; } else { parent->right = nullptr; } delete(root); } } else if (root->val > key) { helper(root->left, root, true, key); } else { helper(root->right, root, false, key); } }};
******************** 迭代。 循环就是个实现题。考的是细心 ********
class Solution { public: TreeNode* deleteNode(TreeNode* root, int key) { TreeNode header; header.left = root; TreeNode * pre = &header; TreeNode * cur = root; bool preLeft = true; while(cur!= nullptr && cur->val != key){ if(cur->val <key){ preLeft = false; pre = cur; cur = cur->right; }else{ preLeft = true; pre = cur; cur = cur->left; } } if(cur!= nullptr){ if(cur->left== nullptr){ if(preLeft) pre->left = cur->right; else pre->right = cur->right; }else if(cur->right == nullptr){ if(preLeft) pre->left = cur->left; else pre->right = cur->left; }else{ // have two children // find the biggest on left children, and detach auto p = cur; auto child = cur->left; while(child->right){ p = child; child = child->right; } cur->val = child->val; if(p==cur){ p->left = child->left; // ERROR made, had assigned nullptr }else{ p->right = child->left; } } } return header.left; } };
ERROR:
就是p->left的时候,以为到了最后一个了,child节点的左右都是Null了, 然而不是的。哎
No comments:
Post a Comment