You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order, and each of their nodes contains a single digit. Add the two numbers and return the sum as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
Example 1:
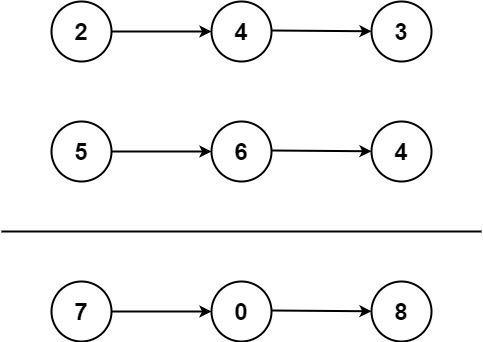
Input: l1 = [2,4,3], l2 = [5,6,4] Output: [7,0,8] Explanation: 342 + 465 = 807.
Example 2:
Input: l1 = [0], l2 = [0] Output: [0]
Example 3:
Input: l1 = [9,9,9,9,9,9,9], l2 = [9,9,9,9] Output: [8,9,9,9,0,0,0,1]
Constraints:
- The number of nodes in each linked list is in the range
[1, 100]
. 0 <= Node.val <= 9
- It is guaranteed that the list represents a number that does not have leading zeros.
A:
Mistakes:
1: 忘记 给l1, l2 也增加runner, --------- 这里其实可以直接用l1,l2当做runner,毕竟java是传引用的。
2: 应该是在计算完毕 sun之后,再update carry的值。
3: 当carry 最后 进一位的时候, 我们copy 其余的产品的时候, 忘了把其也加上。 哎~~~~~
这道题,就是考的细心啊~~~~~~~~~
4: 为什么,( 3+5 )%10 的结果总是1 呢? 搞得carry 变成1 了。 哎 ---------- 计算carry的时候,把r2 写成r1了。
5: 当carry 最后加完了, 还是1 的时候,我们要再生成一个节点。 ╮(╯▽╰)╭, Tiger,你这样不行啊~~~~~~~~~~~~SB错误太多了
--------------------------3 rd pass--------------use only one header, for newly created list header 跟第一遍的写法类似---------------------- 好消息是, 只有几个拼写错误, 不好的是, 还是不能bug free--------------------
/*** Definition for singly-linked list.* struct ListNode {* int val;* ListNode *next;* ListNode() : val(0), next(nullptr) {}* ListNode(int x) : val(x), next(nullptr) {}* ListNode(int x, ListNode *next) : val(x), next(next) {}* };*/class Solution {public:ListNode* addTwoNumbers(ListNode* l1, ListNode* l2) {int carry = 0;ListNode header(0);auto tail = &header;while (carry || l1 || l2) {int a = l1 ? l1->val : 0;int b = l2 ? l2->val : 0;tail->next = new ListNode((a + b + carry) % 10);tail = tail->next;if (l1) {l1 = l1->next;}if (l2) {l2 = l2->next;}carry = (a + b + carry) / 10;}return header.next;}};
-------------------4 th pass---------------recursive way---------------
public class Solution { public ListNode addTwoNumbers(ListNode l1, ListNode l2) { return helper(l1,l2,0); } private ListNode helper(ListNode l1,ListNode l2, int carry){ ListNode curNode; if(l1==null && l2 == null){ return carry == 0?null: new ListNode(carry); }else if(l1 == null){ curNode = new ListNode((carry+l2.val)%10); carry = (carry+l2.val)/10; curNode.next = helper(null,l2.next,carry); }else if(l2 == null){ curNode = new ListNode((carry+l1.val)%10); carry = (carry+l1.val)/10; curNode.next = helper(l1.next,null,carry); }else{ curNode = new ListNode((carry+l1.val+l2.val)%10); carry = (carry+l1.val+l2.val)/10; curNode.next = helper(l1.next,l2.next,carry); } return curNode; } }
----------------------递归调用的 简化版本------------
public class Solution { public ListNode addTwoNumbers(ListNode l1, ListNode l2) { return helper(l1,l2,0); } private ListNode helper(ListNode l1,ListNode l2, int carry){ if(l1==null && l2 == null) return carry == 0?null: new ListNode(carry); int sum = (l1==null?0:l1.val) + (l2==null?0:l2.val)+carry; ListNode curNode = new ListNode((sum)%10); curNode.next = helper(l1==null?null:l1.next,l2==null?null:l2.next,sum/10); return curNode; } }
No comments:
Post a Comment