Given a reference of a node in a connected undirected graph.
Return a deep copy (clone) of the graph.
Each node in the graph contains a val (int
) and a list (List[Node]
) of its neighbors.
class Node { public int val; public List<Node> neighbors; }
Test case format:
For simplicity sake, each node's value is the same as the node's index (1-indexed). For example, the first node with val = 1
, the second node with val = 2
, and so on. The graph is represented in the test case using an adjacency list.
Adjacency list is a collection of unordered lists used to represent a finite graph. Each list describes the set of neighbors of a node in the graph.
The given node will always be the first node with val = 1
. You must return the copy of the given node as a reference to the cloned graph.
Example 1:
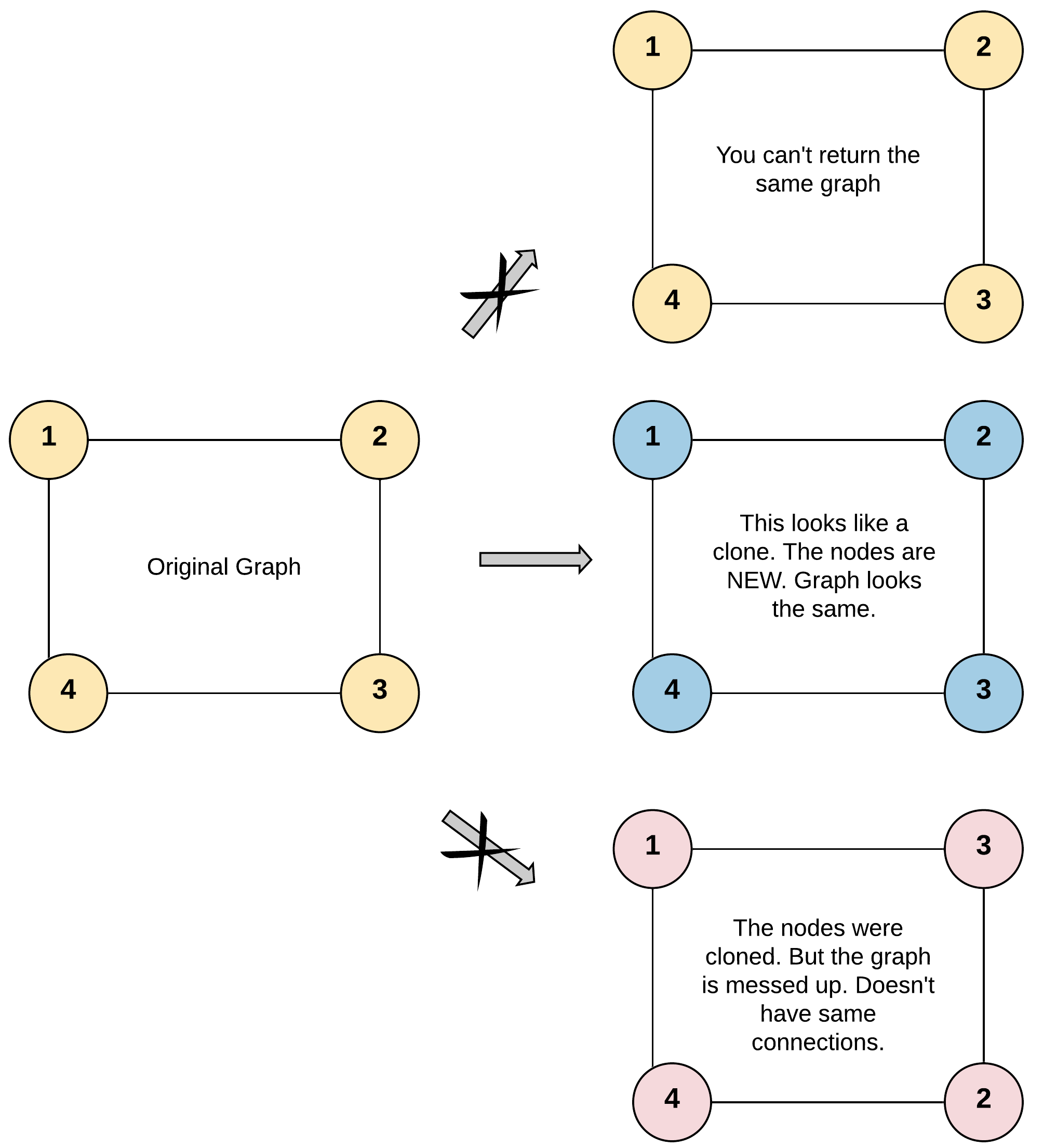
Input: adjList = [[2,4],[1,3],[2,4],[1,3]] Output: [[2,4],[1,3],[2,4],[1,3]] Explanation: There are 4 nodes in the graph. 1st node (val = 1)'s neighbors are 2nd node (val = 2) and 4th node (val = 4). 2nd node (val = 2)'s neighbors are 1st node (val = 1) and 3rd node (val = 3). 3rd node (val = 3)'s neighbors are 2nd node (val = 2) and 4th node (val = 4). 4th node (val = 4)'s neighbors are 1st node (val = 1) and 3rd node (val = 3).
Example 2:
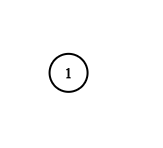
Input: adjList = [[]] Output: [[]] Explanation: Note that the input contains one empty list. The graph consists of only one node with val = 1 and it does not have any neighbors.
Example 3:
Input: adjList = [] Output: [] Explanation: This an empty graph, it does not have any nodes.
Example 4:
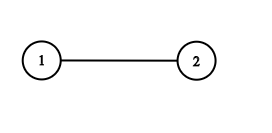
Input: adjList = [[2],[1]] Output: [[2],[1]]
Constraints:
1 <= Node.val <= 100
Node.val
is unique for each node.- Number of Nodes will not exceed 100.
- There is no repeated edges and no self-loops in the graph.
- The Graph is connected and all nodes can be visited starting from the given node.
经典DFS题目
这个题目的难点在于 要copy 邻居,导致需要所有的邻居create之后,才能add neighbors, 因此,我们需要先dfs ,再add neighbors
/*// Definition for a Node.class Node {public:int val;vector<Node*> neighbors;Node() {val = 0;neighbors = vector<Node*>();}Node(int _val) {val = _val;neighbors = vector<Node*>();}Node(int _val, vector<Node*> _neighbors) {val = _val;neighbors = _neighbors;}};*/class Solution {public:Node* cloneGraph(Node* node) {if (!node)return nullptr;unordered_map<Node*, Node*> map;dfs_clone_val(node, map);return map[node];}private:void dfs_clone_val(Node* node, unordered_map<Node*, Node*>& map) {if (map.find(node) != map.end())return;map[node] = new Node(node->val);for (auto kk : node->neighbors)dfs_clone_val(kk, map);for (auto kk : node->neighbors)map[node]->neighbors.push_back(map[kk]);}};
Mistakes:
1: when creating a new node, we should add it into the HashMap immediately, ------------rather than add into hashMap after visiting©ing his neighbors
2:总是miss掉很多节点的 问题找到了------------------在create new node的时候,我们也顺便create 了他们的neighbor, 但是, 这些neighbor的 neighbor,并没有被copy过来。
因此,我们需要check一下。
5: 有个问题,就是说。当我们给定一个node,查询其neighbor的时候,我们只需要建立(或者找到) 新建的,具有相同label的点即可。(这个时候,不需要建立新的点的neighbor)等以后,我们真正visit 其节点的时候,再update neighbor 链表。
-------------------------第3遍----------BFS------------------
唉,这次,3个月没写code,完全忘记了。
唯一想明白的是,由于neighbor的问题, 我们走两遍,第一遍先create all the new nodes, and in the second iteration, we link all the neighbors.
这道题的关键点,在于, 在第二遍遍历的时候,要在每个neighbor List中,加入Set,因为有可能会有2条neighbor指向同一个目标节点。 因此先加入的话,会有重复----
Mistakes:/*// Definition for a Node.class Node {public:int val;vector<Node*> neighbors;Node() {val = 0;neighbors = vector<Node*>();}Node(int _val) {val = _val;neighbors = vector<Node*>();}Node(int _val, vector<Node*> _neighbors) {val = _val;neighbors = _neighbors;}};*/class Solution {public:Node* cloneGraph(Node* node) {if (node == nullptr)return nullptr;unordered_map<Node*, Node*> M;queue<Node*> Q;Q.push(node);while (not Q.empty()) {auto cur = Q.front();Q.pop();if (M.find(cur) == M.end()) { // if NOT already clonedM[cur] = new Node(cur->val);for (auto nei : cur->neighbors) {Q.push(nei);}}}// setup the neighborsunordered_set<Node*> visited;Q.push(node);while (not Q.empty()) {auto cur = Q.front();Q.pop();if (visited.find(cur) == visited.end()) {visited.insert(cur);for (auto nei : cur->neighbors) {M[cur]->neighbors.push_back(M[nei]);Q.push(nei);}}}return M[node];}};----------再仔细考虑,其实可以一个循环走完的。巧妙在Queue中加的都是还没有copy 边的Node*,因此可以直接操作class Solution {public:Node* cloneGraph(Node* node) {if (node == nullptr)return nullptr;unordered_map<Node*, Node*> M;queue<Node*> Q;Q.push(node);M[node] = new Node(node->val);while (not Q.empty()) {auto old = Q.front();Q.pop();for(auto & nei : old->neighbors){if(M.find(nei) == M.end()){M[nei] = new Node(nei->val);Q.push(nei); // contains only newly created node}M[old]->neighbors.push_back(M[nei]);}}return M[node];}};
No comments:
Post a Comment