Given an m x n
matrix
, return all elements of the matrix
in spiral order.
Example 1:
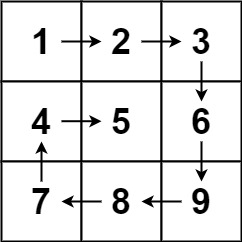
Input: matrix = [[1,2,3],[4,5,6],[7,8,9]] Output: [1,2,3,6,9,8,7,4,5]
Example 2:
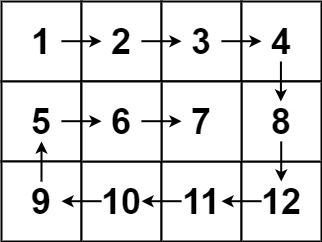
Input: matrix = [[1,2,3,4],[5,6,7,8],[9,10,11,12]] Output: [1,2,3,4,8,12,11,10,9,5,6,7]
Constraints:
m == matrix.length
n == matrix[i].length
1 <= m, n <= 10
-100 <= matrix[i][j] <= 100
A:
一层层地剥开罢, 按照上->右->下->左的顺序加入即可。注意,当只有一行,一列的情况。(某一行,或某一列尽量取多的值。 同时, 在检查下面行,左边列的时候,要检查是否本行/列 已经加过。
class Solution {public:vector<int> spiralOrder(vector<vector<int>>& matrix) {vector<int> res;int m = matrix.size(), n = matrix[0].size();int layer = 0; // layer starting from 0,while (layer <= m - 1 - layer && layer <= n - 1 - layer) {int r0 = layer, c0 = layer;int r1 = layer, c1 = n - 1 - layer;int r2 = m - 1 - layer, c2 = n - 1 - layer;int r3 = m - 1 - layer, c3 = layer;// top row, (gready first)for (int j = c0; j <= c1; j++) {res.push_back(matrix[r0][j]);}// right columnfor (int i = r1+1; i <= r2; i++) {res.push_back(matrix[i][c1]);}// bottom rowif (r2 > r0) {for (int j = c2 - 1; j >= c3; j--) {res.push_back(matrix[r2][j]);}}// left columnif (c3 < c2) {for (int i = r3 - 1; i > r0; i--) {res.push_back(matrix[i][c3]);}}layer++;}return res;}};
Mistakes:
1: 由于我们每个边都是取了开头,没有取最后一个。 导致,当只有一个点的时候,我们没能取出来。
解决方法: 把upper row,全部取出来。
2; 当只有一行的时候, 我们取了两遍, 而这里,我们应该是先比较行数和列数,是否相同的。
3: 当只有一列的时候, 类似于第一个错误,我们应该在某一列, 尽量取多的值。
i. e. right col 第一个不取,但是取最后一个。
No comments:
Post a Comment