Given the head
of a sorted linked list, delete all duplicates such that each element appears only once. Return the linked list sorted as well.
Example 1:
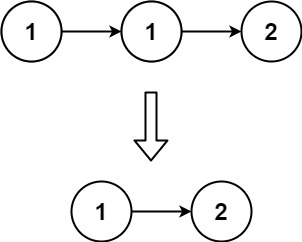
Input: head = [1,1,2] Output: [1,2]
Example 2:
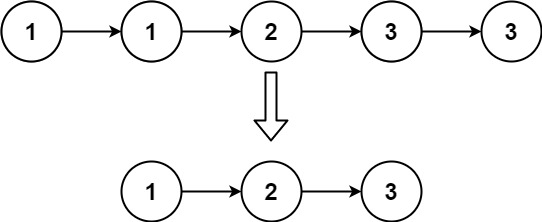
Input: head = [1,1,2,3,3] Output: [1,2,3]
Constraints:
- The number of nodes in the list is in the range
[0, 300]
. -100 <= Node.val <= 100
- The list is guaranteed to be sorted in ascending order.
------------------------------------------3 rd Pass ---------------------------
错误: 刚开始,while内部,又用了个while,写成了/*** Definition for singly-linked list.* struct ListNode {* int val;* ListNode *next;* ListNode() : val(0), next(nullptr) {}* ListNode(int x) : val(x), next(nullptr) {}* ListNode(int x, ListNode *next) : val(x), next(next) {}* };*/class Solution {public:ListNode* deleteDuplicates(ListNode* head) {auto pre = head;while(pre && pre->next){auto cur = pre->next;if(cur->val == pre->val){ // remove current nodepre->next = cur->next;}else{pre = cur;}}return head;}};
while (tail != null && tail.next != null) { while (tail.val == tail.next.val) {......delete the next node........}可是这样的话,就会导致 tail.next已经指向了null指针。
--------------------------------1st Pass -------------------------------------
就是两个指针,看是否相同。
public class Solution { public ListNode deleteDuplicates(ListNode head) { ListNode p1, p2; // p1 is the current node, p2 is his next neighbor if(head ==null || head.next == null){ return head; } //now we have at least 2 Nodes in the list p1=head; p2 = head.next; while(p2!= null){ if(p2.val == p1.val){ // delete p2, p1.next = p2.next; p2 = p1.next; }else{ p1= p2; p2 = p1.next; } } return head; } }
No comments:
Post a Comment